In this course, we will be taking a look at how to make use f the activity indicator in react native.
Step 1: App
your App component will be used to import and show our ActivityIndicator.
App.js
import React from 'react' import ActivityIndicatorExample from './activity_indicator_example.js' const Home = () => { return ( <ActivityIndicatorExample /> ) } export default Home
Step 2: ActivityIndicatorExample
Animating property is a Boolean which is used for showing the activity indicator. The latter closes six seconds after the component is mounted. This is done using the closeActivityIndicator() function.
activity_indicator_example.js
import React, { Component } from 'react'; import { ActivityIndicator, View, Text, TouchableOpacity, StyleSheet } from 'react-native'; class ActivityIndicatorExample extends Component { state = { animating: true } closeActivityIndicator = () => setTimeout(() => this.setState({ animating: false }), 60000) componentDidMount = () => this.closeActivityIndicator() render() { const animating = this.state.animating return ( <View style = {styles.container}> <ActivityIndicator animating = {animating} color = '#bc2b78' size = "large" style = {styles.activityIndicator}/> </View> ) } } export default ActivityIndicatorExample const styles = StyleSheet.create ({ container: { flex: 1, justifyContent: 'center', alignItems: 'center', marginTop: 70 }, activityIndicator: { flex: 1, justifyContent: 'center', alignItems: 'center', height: 80 } })
When we run the app, we will see the loader on screen. It will disappear after six seconds.
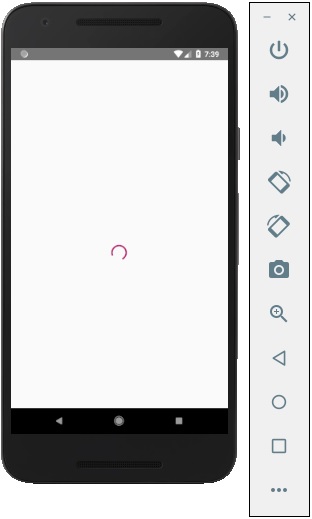